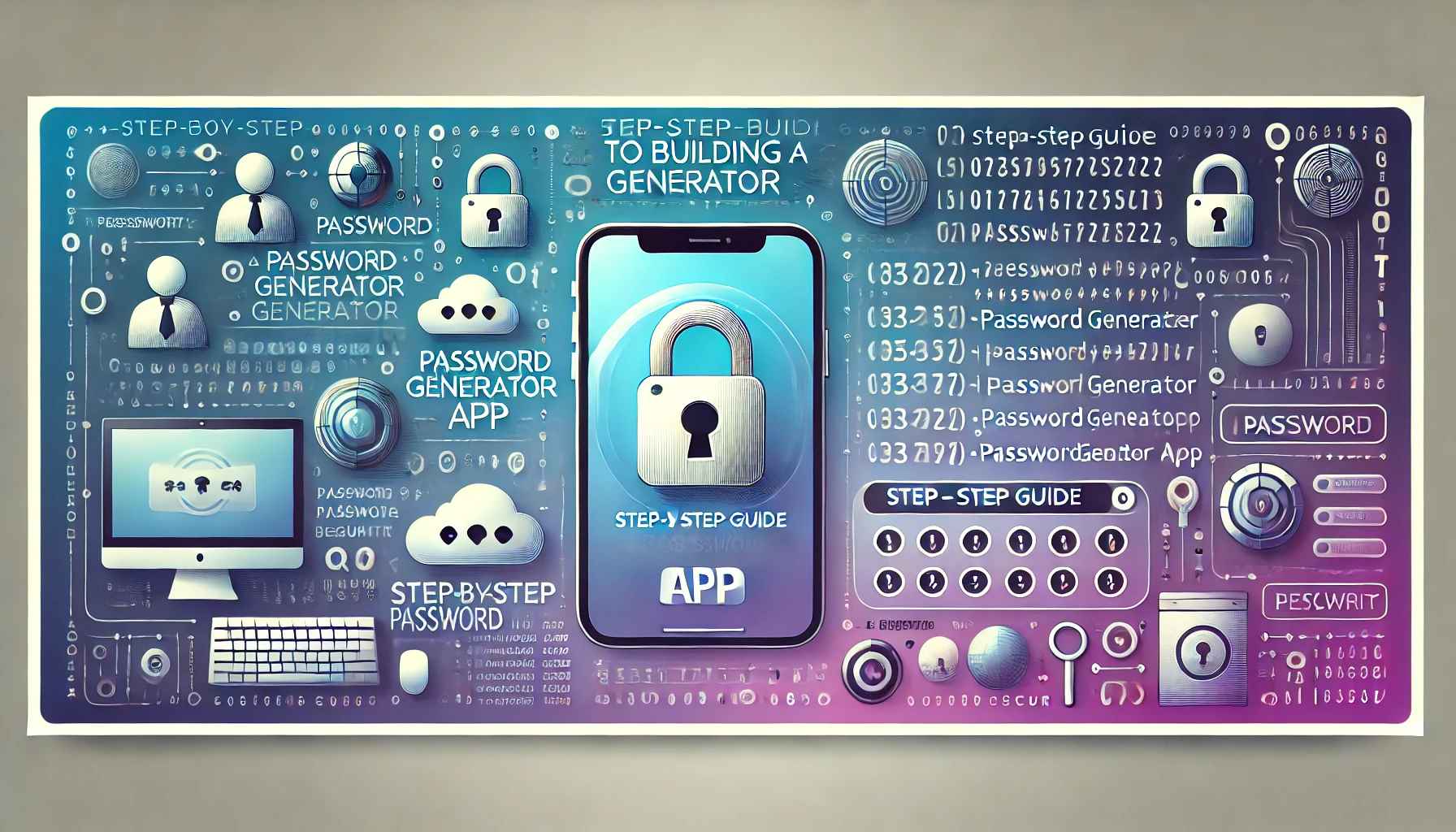
Creating a Password Generator App is a great way to practice programming skills and build a handy tool for generating secure passwords. This guide will walk you through the necessary steps, explaining each phase to make it clear and easy to follow.
Why Create a Password Generator App?
In today’s digital world, passwords are critical for protecting online information. A password generator app allows you to create strong, unique passwords that are difficult to guess or hack, which adds a layer of security to your accounts. By building your app, you gain control over customization and can always generate secure passwords when needed.
Project Overview
This Password Generator App will:
- Allow users to specify the password length.
- Offer options including uppercase, lowercase, numbers, and symbols.
- Generate a random password based on the user’s selected options.
Steps to Build the Password Generator App
Step 1: Define the Purpose and Requirements
First, decide what the app will do and what features it should have. Here are the basic requirements for a password generator:
- A way for users to input their preferences for password length and character types (including uppercase letters, numbers, and symbols).
- A function to create a random password based on these inputs.
- They are displaying the generated password so users can copy it easily.
Step 2: Plan the Character Sets
A password is a mix of characters, so plan which types of characters you want the app to use:
- Lowercase Letters: a, b, c, …, z
- Uppercase Letters: A, B, C, …, Z
- Numbers: 0, 1, 2, …, 9
- Symbols: Characters like @, #, $, %, etc., that make passwords more secure.
The app will combine these character sets based on user preferences to generate a password.
Step 3: Design the User Interface (UI)
Consider how you want users to interact with your app. For a simple version, the UI could have:
- An input for password length.
- Checkboxes or toggles for including uppercase letters, numbers, and symbols.
- A “Generate Password” button to trigger the creation of a new password.
- A display area to show the generated password.
If you’re comfortable with graphical design, you could add this using a simple GUI (Graphical User Interface) library or even a web-based interface for added ease.
Step 4: Build the Password Generation Logic
The core function of this app is to generate a password based on the user’s preferences. Here’s the logic in simple terms:
1. Start with a Default Character Set:
Begin with lowercase letters as the base option since they’re required for a standard password.
2. Add Additional Characters:
If the user wants uppercase letters, numbers, or symbols, add them to the pool of characters the app will use.
3. Generate the Password:
Randomly pick characters from this pool until you reach the desired password length.
4. Display the Result:
Show the generated password so the user can copy it.
Step 5: Handle User Input
Let the user enter or select their preferences for:
- Password Length: An input for how many characters they want (e.g., 8-20 characters).
- Character Types: Options for including uppercase letters, numbers, and symbols.
When these options are set, the app can build the password with the appropriate level of complexity based on the selections.
Step 6: Add Error Handling
To improve the user experience, handle potential errors. For example:
Password Length:
Ensure the password length is realistic. Generally, passwords should be at least 8 characters long for security.
User Choices:
If no options are selected (like numbers or symbols), inform the user to add more character types for better security.
Adding these checks ensures the app runs smoothly and guides users to create strong, secure passwords.
Step 7: Test the App
Once the basic functionality is complete, test your app thoroughly:
- Try different lengths and character combinations.
- Ensure that it generates a unique password each time.
- Verify that the passwords match the user’s selected preferences (length and character types).
Testing helps to find any bugs or areas for improvement, ensuring the app performs as expected.
Step 8: Optional GUI Enhancement
If you’d like to make the app more user-friendly, consider adding a graphical interface. A GUI allows users to select options with buttons and checkboxes, making the app more visually appealing and easier to navigate. This can be done with tools for creating simple interfaces, either through desktop GUI frameworks or a web-based approach.
Final Thoughts
A Password Generator App is a great project to strengthen programming skills and understand the logic of creating randomized outputs. By following these steps, you’ll have a functional and customizable app that generates strong passwords and enhances online security.
With some creativity, you can extend this project by adding features like:
- Password Storage: Store generated passwords securely.
- Customization Options: Allow users to exclude specific characters or set custom patterns.
- Mobile Compatibility: Turn your app into a mobile-friendly tool for even easier access.
Enjoy building your app, and remember, each step you complete will bring you closer to mastering core programming concepts. Happy coding!